includes()方法判断是否包含某一元素,返回true或false表示是否包含元素,对NaN一样有效
filter()方法用于把Array的某些元素过滤掉,filter()把传入的函数依次作用于每个元素,然后根据返回值是true还是false决定保留还是丢弃该元素:生成新的数组
一、单选
<template>
<ul>
<li v-for="(city, index) in cityList" :key="index" :class="{ checked: index === checkedIndex }"
@click="changChecked(index)">
{{ city }}
</li>
</ul>
</template>
<script>
export default {
name: 'Home',
data() {
return {
checkedIndex: 0,
cityList: ['上海', '北京', '广州', '西安']
}
},
methods: {
changChecked(index) {
this.checkedIndex = index
}
}
}
</script>
<style lang="scss" scoped>
ul {
padding: 0;
li {
display: inline-block;
border: 1px solid #000;
border-radius: 8px;
margin-left: 5px;
width: 80px;
height: 50px;
text-align: center;
line-height: 50px;
cursor: pointer;
transition: all 0.3s linear;
&.checked {
border: 1px solid #fff;
color: #fff;
background-color: #0cf;
}
&:hover {
border: 1px solid #fff;
color: #fff;
background-color: #0cf;
}
}
}
</style>
效果如图所示:

二、多选
方法一:
思路
选中标识值为数组,标识值包含当前对像标识时(本例使用索引),给所在标签添加选中样式;点击标签时,若不包含选中标识,添加到数组;否则从数组从移除。
<template>
<ul>
<li v-for="(city, index) in cityList" :key="index"
:class="{ checked: checkedList.includes(index) }"
@click="changChecked(index)"> {{ city }} </li>
</ul>
</template>
<script>
export default {
name: 'Home2',
data() {
return {
checkedList: [],
cityList: ['上海', '北京', '广州', '西安'],
}
},
methods: {
changChecked(index) {
if (this.checkedList.includes(index)) {
this.checkedList = this.checkedList.filter(item => {
return item !== index
})
} else {
this.checkedList.push(index)
}
}
}
}
</script>
<style lang="scss" scoped>
ul {
padding: 0;
list-style: none;
li {
display: inline-block;
margin-left: 5px;
width: 50px;
height: 30px;
text-align: center;
line-height: 30px;
cursor: pointer;
&::before {
display: inline-block;
margin-right: 2px;
border: 1px solid #000;
width: 10px;
height: 10px;
line-height: 10px;
content: "";
transition: all 0.3s linear;
}
&.checked {
color: #0CF;
}
&.checked::before {
border: 1px solid #fff;
background-color: #0CF;
}
}
}
</style>
方法二:
思路
通过引入辅助变量实现。每个对象添加一个是否选中标识,若标识为 true
,给所在标签添加选中样式;点击标签时,取反所在对象标识。
<template>
<ul>
<li v-for="(item, index) in cityList" :key="index" :class="{ checked: item.checked }"
@click="changChecked(index)">
{{ item.city }}
</li>
</ul>
</template>
<script>
export default {
name: 'Home3',
data() {
return {
cityList: [{
city: '上海',
checked: false
},
{
city: '北京',
checked: false
},
{
city: '广州',
checked: false
},
{
city: '西安',
checked: false
}
]
}
},
methods: {
changChecked(index) {
this.cityList[index].checked = !this.cityList[index].checked
}
}
}
</script>
<style lang="scss" scoped>
ul {
padding: 0;
list-style: none;
li {
display: inline-block;
margin-left: 5px;
width: 50px;
height: 30px;
text-align: center;
line-height: 30px;
cursor: pointer;
&::before {
display: inline-block;
margin-right: 2px;
border: 1px solid #000;
width: 10px;
height: 10px;
line-height: 10px;
content: "";
transition: all 0.3s linear;
}
&.checked {
color: #0CF;
}
&.checked::before {
border: 1px solid #fff;
background-color: #0CF;
}
}
}
</style>
效果如图所示:
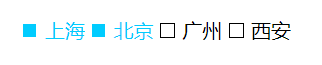